PHP Error Handling: Mastering Exception and Error Challenges
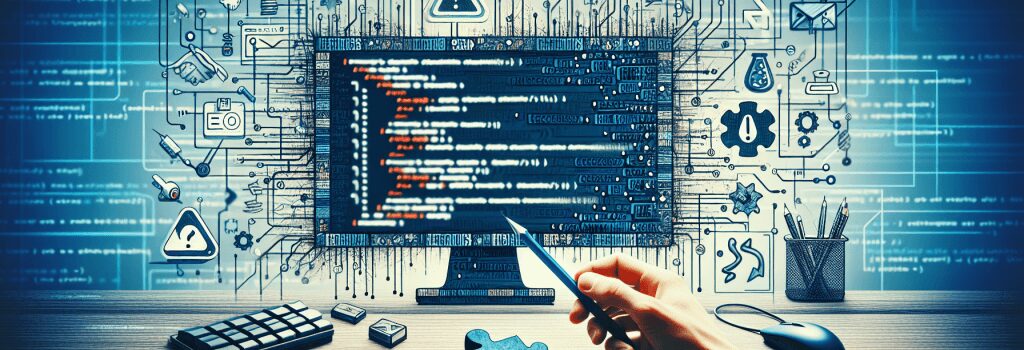
PHP, a server-side scripting language, plays a crucial role in web development, powering everything from small websites to huge web applications like Facebook and WordPress. As a budding web developer, mastering PHP is essential, but equally important is understanding how to handle errors and exceptions efficiently. This article delves into PHP error handling, an essential skill for developers looking to ensure smooth and reliable web application performance.
Understanding PHP Error Handling
Error handling in PHP is a method to deal with runtime errors, allowing a script to continue running even if something goes wrong. This is crucial in web development, as it helps in identifying, managing, and correcting errors without crashing the application or website.
Types of Errors in PHP
Before diving into error handling, let’s understand the different types of errors in PHP:
– Syntax Errors: Errors in the code syntax, such as missing semicolons or misspelled keywords.
– Warning Errors: Non-critical errors that do not stop script execution, like including a missing file.
– Fatal Errors: Critical errors that halt script execution, such as calling undefined functions.
– Notice Errors: Minor errors that don’t affect script execution, often related to coding standards.
Exception Handling in PHP
Exception handling is a more advanced error handling method introduced in PHP 5. Exceptions are used to signal errors that can be caught and handled by the developer.
Try and Catch Blocks
The try-catch block is the cornerstone of exception handling in PHP. It works by trying to execute a block of code and catching any exceptions that are thrown.
The Exception Class
PHP provides a built-in Exception class, which can be extended to create custom exceptions. This class contains methods like ;getMessage()>, ;getCode()>, and ;getFile()>, which provide details about the exception.
Error Reporting in PHP
PHP allows you to configure error reporting levels via the ;error_reporting()> function. This function is essential for development as it can be used to show all errors for debugging. It’s advisable to set a lower error reporting level in production to avoid exposing sensitive information.
Custom Error Handlers
PHP allows the creation of custom error handlers using the ;set_error_handler()> function. This can be particularly useful for converting errors into exceptions, logging errors, or displaying user-friendly error messages.
Best Practices for PHP Error Handling
– Use exceptions for error handling in object-oriented PHP.
– Leverage try-catch blocks for code sections that may throw exceptions.
– Implement a global exception and error handler to catch uncaught exceptions and errors.
– Log errors for later analysis to improve the application.
– Always validate user input to prevent common errors.
Conclusion
Mastering error handling in PHP is critical for developing robust and reliable web applications. By understanding and implementing the techniques discussed in this article, such as exception handling, custom error handling, and configuring error reporting, developers can ensure their PHP applications can gracefully handle errors and provide a better user experience.
By embracing these error and exception handling challenges in PHP, you’ll be well on your way to becoming a proficient web developer, capable of crafting resilient and efficient web applications. Happy coding!