Advanced PHP: Object-Oriented Programming and MVC Concepts
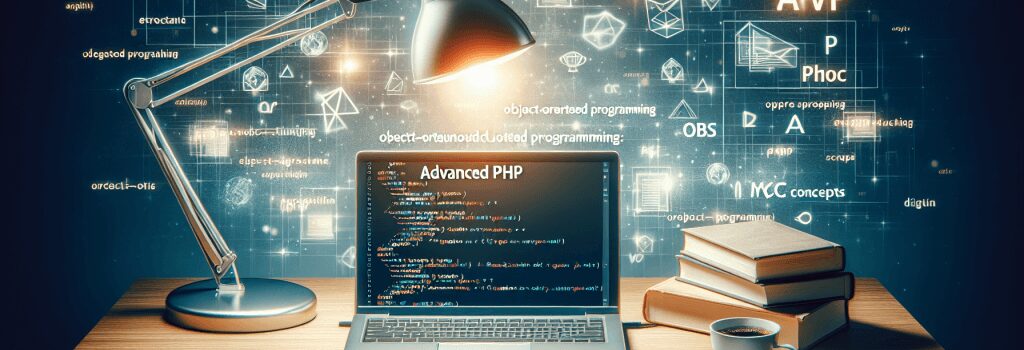
—
Unlocking the Power of Advanced PHP: Mastering Object-Oriented Programming and MVC Concepts
In the journey to becoming a competent web developer, mastering PHP is a crucial step. PHP, a server-side scripting language, is not only foundational for building dynamic and interactive web applications but also critical for leveraging WordPress capabilities. This guide delves into Advanced PHP, focusing on Object-Oriented Programming (OOP) and Model-View-Controller (MVC) concepts. These paradigms elevate your coding skills and enable you to create more efficient, maintainable, and scalable applications.
Understanding Object-Oriented Programming (OOP)
The Basics of OOP
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of “objects”, which can contain data and code: data in the form of fields (often known as attributes or properties), and code, in the form of procedures (often known as methods).
Key Principles of OOP
– Encapsulation: This principle states that all data (attributes) and methods (functions) are bundled within the object, ensuring a clear modular structure for applications.
– Inheritance: This allows a new class to inherit the properties and methods of another class, promoting code reuse and efficiency.
– Polymorphism: It enables objects of different classes to be treated as objects of a common super class, mainly for implementing shared functionalities in varied ways.
– Abstraction: This concept hides complex implementation details and exposes only the necessary part of the object to the outside world.
Understanding and implementing these principles in your PHP projects can significantly improve the robustness and flexibility of your applications.
Exploring MVC Architecture
What is MVC?
Model-View-Controller (MVC) is a design pattern that separates an application into three interconnected components: the model, the view, and the controller. This promotes organized coding and simplifies the development process.
Components of MVC
– Model: Represents the data and business logic layer of the application. It manages the data and rules of the application.
– View: Represents the UI layer of the application. It displays the data to the user, based on the models.
– Controller: Acts as an interface between Model and View components, processing all the business logic and incoming requests, manipulating data using the Model, and interacting with the Views to render the final output.
Benefits of MVC in PHP
– Separation of Concerns: MVC inherently promotes the separation of the front-end and the back-end, which enhances code clarity and scalability.
– Reusability and Modularity: Components in an MVC application can be independently developed and reused, significantly speeding up the development process.
– Improved Collaboration: MVC architecture allows developers to work on the model, controller, and views independently, which can be particularly beneficial in teams.
Implementing OOP and MVC in PHP
Practical Tips
1. Start Small: Begin by encapsulating your PHP code into classes (if you haven’t already) and understand how objects work.
2. Refactor Gradually: Integrate OOP principles into your existing projects incrementally. For example, convert a WordPress plugin to a class-based structure.
3. MVC Frameworks: Consider using PHP MVC frameworks like Laravel, Symfony, or CodeIgniter. These frameworks provide a robust structure for developing complex applications efficiently.
Beyond Basics
Advanced PHP development, particularly with OOP and MVC, requires ongoing learning and practice. Engage with community forums, explore open-source projects, and never stop experimenting with new techniques and frameworks.
Conclusion
Advancing your skills in PHP with Object-Oriented Programming and MVC concepts is a transformative step in your web development career. These paradigms not only optimize your code but also make it more maintainable and scalable, thus enabling you to build complex web applications with efficiency and ease. Embrace the journey of mastering advanced PHP, for it is replete with opportunities for growth and innovation.
—
By integrating strategic SEO keywords related to “Advanced PHP,” “Object-Oriented Programming,” “MVC Concepts,” and “Web Development” throughout the article, this content is optimized to attract readers aiming to deepen their understanding of PHP in web development.