Advanced Techniques for PHP String and Array Debugging
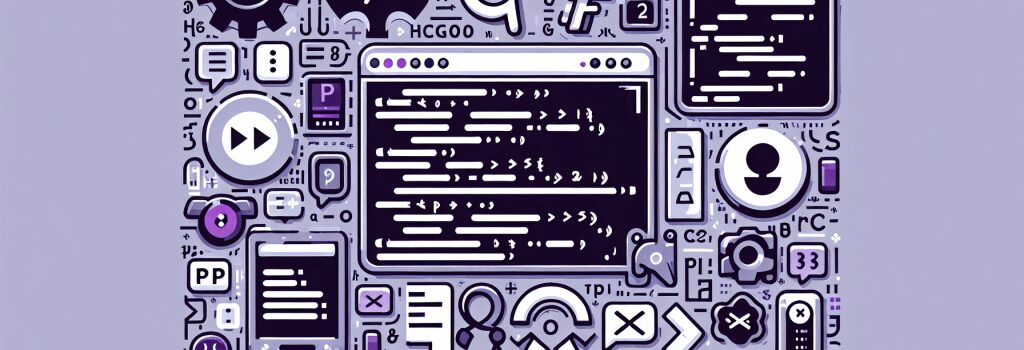
Introduction to Debugging PHP Strings and Arrays
Debugging is a critical skill for any developer, and when working with PHP, mastering the debugging of strings and arrays is fundamental. Strings and arrays are two of the most commonly used data types in PHP, serving as the backbone of data manipulation and representation in many web applications. Whether you’re an aspiring developer or looking to sharpen your PHP skills, understanding advanced techniques for debugging strings and arrays is essential.
Understanding the Basics of PHP Strings and Arrays
Before diving into advanced debugging techniques, it’s important to have a solid understanding of what strings and arrays are in PHP. A string is essentially a sequence of characters, while an array is a data structure that holds multiple values in a single variable.
Debugging PHP Strings
Working with var_dump() and print_r()
When debugging strings, functions like ;var_dump()> and ;print_r()> are invaluable. These functions allow you to output the content of a string, providing insights into its structure and contents.
– Using var_dump(): This function displays the data type and value of a given variable. When debugging strings, ;var_dump()> can help identify issues related to string length or unexpected characters.
– Applying print_r(): While similar to ;var_dump()>, ;print_r()> is often more readable, making it ideal for debugging more complex string content.
String Manipulation Functions
PHP offers a wide range of string manipulation functions that can be useful in debugging. Functions like ;strlen()>, ;strpos()>, and ;str_replace()> allow you to analyze and modify strings, helping identify and resolve issues.
Debugging PHP Arrays
Arrays can be more complex to debug due to their potential size and depth. Advanced techniques often involve analyzing the structure and contents of an array in detail.
Leveraging var_dump() and print_r()
Just as with strings, ;var_dump()> and ;print_r()> are essential for debugging arrays. They allow you to visualize the entire array, including nested arrays, giving insights into its structure.
Understanding array Functions
PHP provides a rich set of functions for manipulating arrays, which can be powerful tools in debugging. Functions like ;count()>, ;array_merge()>, and ;array_diff()> can help you understand the composition of your arrays and identify any discrepancies.
Utilizing foreach for Inspection
The ;foreach> loop is particularly useful for debugging arrays. It allows you to iterate over each element of an array, applying custom debugging logic or simply printing out values for inspection.
Debugging with Xdebug
While manual debugging techniques are effective, leveraging a tool like Xdebug can take your debugging capabilities to the next level. Xdebug is a PHP extension that provides debugging and profiling capabilities.
– Setting Breakpoints: With Xdebug, you can set breakpoints in your code, pausing execution to inspect the state of strings and arrays at specific points in your application.
– Step-through Debugging: This feature allows you to execute your PHP code one line at a time, inspecting the state of variables along the way.
Conclusion
Mastering the art of debugging PHP strings and arrays is crucial for anyone aspiring to become a skilled web developer. By combining manual techniques with powerful tools like Xdebug, you can efficiently identify and resolve issues in your PHP code. Remember, practice is key to becoming proficient in debugging, so take the time to apply these techniques in your projects.