JavaScript Promises and Async/Await: Asynchronous Programming Challenges
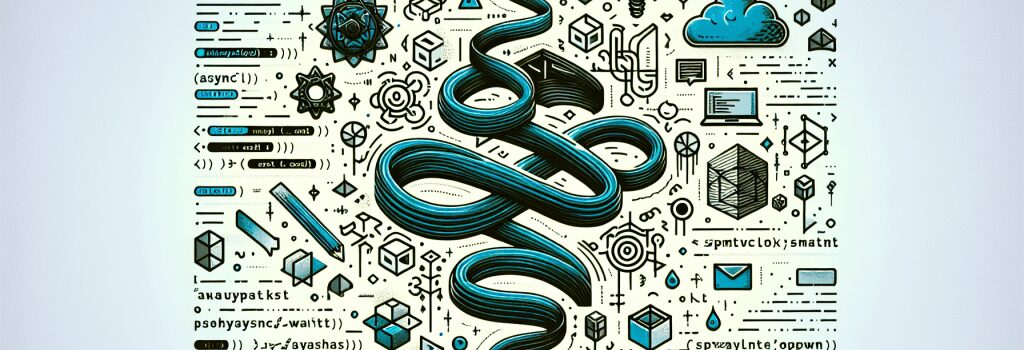
Embracing Asynchronous JavaScript: Mastering Promises and Async/Await
In the rapidly evolving world of web development, mastering asynchronous programming is a crucial step toward building efficient and responsive applications. JavaScript, with its promises and async/await syntax, offers a powerful set of tools for handling asynchronous operations, making it essential for web developers to understand and apply these concepts effectively. This article dives into the world of asynchronous programming in JavaScript, covering the basics of promises and async/await, and providing practical challenges to sharpen your skills.
Understanding JavaScript Promises
A Promise in JavaScript represents the eventual completion (or failure) of an asynchronous operation and its resulting value. Think of it as a placeholder for a value that isn’t known immediately but will be resolved eventually.
<h4>Key Features of Promises:– States: A promise can be in one of three states: pending, fulfilled, or rejected.
– Chaining: Promises can be chained with ;.then()> for success scenarios, and ;.catch()> for handling errors.
– Creating Promises: You create a promise by using the ;new Promise()> constructor which takes a function as an argument. This function has two parameters: ;resolve> and ;reject>, which are used to resolve or reject the promise, respectively.
Diving Into Async/Await
;async> and ;await> are extensions of promises in JavaScript that simplify working with asynchronous functions. By marking a function with ;async>, you promise to return a value. The ;await> keyword, used inside ;async> functions, pauses the execution until the promise is resolved, resulting in cleaner and more intuitive code.
<h4>How to Utilize Async/Await:– Mark functions with ;async> when you declare them.
– Use ;await> before calling any function that returns a Promise.
– Try-Catch blocks can be used within ;async> functions to catch any errors from awaited promises.
Challenges to Enhance Your Skills
To master asynchronous programming with JavaScript promises and async/await, engaging in practical challenges is invaluable. Here are some coding exercises designed to enhance your understanding:
1. Fetch API Challenge: Use the Fetch API to retrieve data from a public API and display the results on your webpage. Implement error handling using promises and then refactor the same challenge using async/await for cleaner code.
2. Chaining Promises: Create a series of functions that return promises and chain them together using ;.then()> and ;.catch()> to understand how sequential asynchronous operations can be handled.
3. Async/Await with Looping: Use ;async>/;await> within a loop to handle multiple asynchronous operations in sequence. This challenge helps in understanding how to manage dynamic collections of promises.
4. Error Handling: Practice using ;try-catch> blocks within async functions to handle errors gracefully, ensuring your applications are robust and user-friendly.
Conclusion
The ability to handle asynchronous operations using promises and async/await is essential for modern web developers. By understanding the principles outlined in this article and putting them to practice through coding challenges, you’ll be well on your way to mastering asynchronous programming in JavaScript. This knowledge not only enhances your coding skill set but also significantly improves the performance and user experience of the web applications you develop. Happy coding!