PHP and APIs: Fetching, Processing, and Displaying Third-Party Data
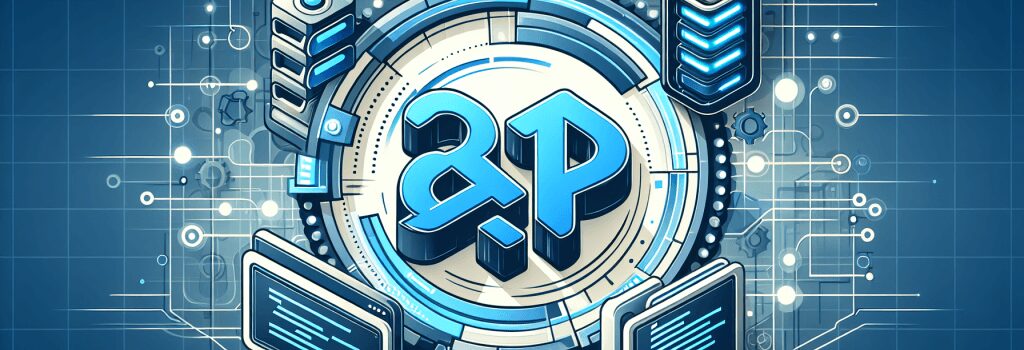
Introduction
Hello there! You have landed on chapter 308 ‘PHP and APIs: Fetching, Processing, and Displaying Third-Party Data’. Before we dive deep into the world of PHP programming with APIs, take a moment and pat yourself on the back. There, doesn’t that feel good? That’s right – you’re learning important stuff, and every single lesson counts towards your goals. And, as we all know, confidence in PHP programming can help you code nearly as well as a cup of coffee can.
What is an API?
Alright, let’s cut to the chase. Have you ever wondered how your tiny smartphone can tell you what the weather is in Bamako, Mali, in near real-time? Or how your favourite travel app finds the cheapest flight to Antarctica (hot destination, right?) among thousands of possible itineraries? The answer, my friend, is not blowing in the wind. It’s with APIs.
API, or Application Programming Interface, serves as a bridge between different software applications, enabling them to communicate and exchange data. As PHP developers, APIs will open up a world of possibilities, allowing our applications to be smarter, well-informed, and more useful to the users. In other words, as a PHP developer, mastering the use of APIs is as important as Batman learning how to throw a Bat-boomerang.
Hello!! Anybody Home?? Making API Calls with PHP.
Now let’s figure out how to use PHP to knock on an API’s door and request data. You can compare an API call to ordering a pizza over the phone – you specify your request (like extra cheese, hold the pineapple), and in return, you receive delicious, hot, and potentially life-altering pizza. Only in this case, instead of pizza, we get valuable data.
;$ch = curl_init(‘https://api.somesite.com/resource?id=234’);>
;curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);>
;$data = curl_exec($ch);>
;curl_close($ch);>
This is a simple example of how you would make an API call with PHP using the cURL library. You can tell by the lack of pineapples. The returned data ($data) would usually be in JSON or XML format.
Process It Like a Pro
Processing the data depends a lot on how it comes back, but in most cases, it’s going to be JSON. Don’t worry; PHP has you covered like an overly attached girlfriend with its json_decode function.
;$processed_data = json_decode($data);>
Boom! You’ve got processed data! Now you can arrange it to your liking and utilize it to enhance your application.
Displaying the Data
When you have received and processed the API data, your PHP application is now analogous to a dog who caught the mailman’s truck – what do we do now? Well, we display it of course! Depending on your application, this can be as simple as generating some HTML with echo or print.
Let’s say we obtained some weather data:
;echo “The temperature in Bamako is “.$processed_data->main->temp.” degrees Celsius.”;>
And voila! You just fetched, processed, and displayed third-party data with PHP and APIs!
Believe me when I say, you are now part of an elite group of PHP developers who can fetch and serve data like a world-class tennis player. So, keep up the good work, pat yourself on the back one more time, and let’s continue on this amazing journey!
In the next chapter, we’ll cover ‘PHP and Error Handling: The Art of Not Breaking Things’. Remember, being a great PHP programmer is like being a cat – even when you fall, always land on your feet! Let’s stay curious, inspired, and passionate about PHP. Until next time, happy coding!
#A PHP Developer’s Journey | END OF CHAPTER 308