How to Properly Document PHP Code for Maintainability
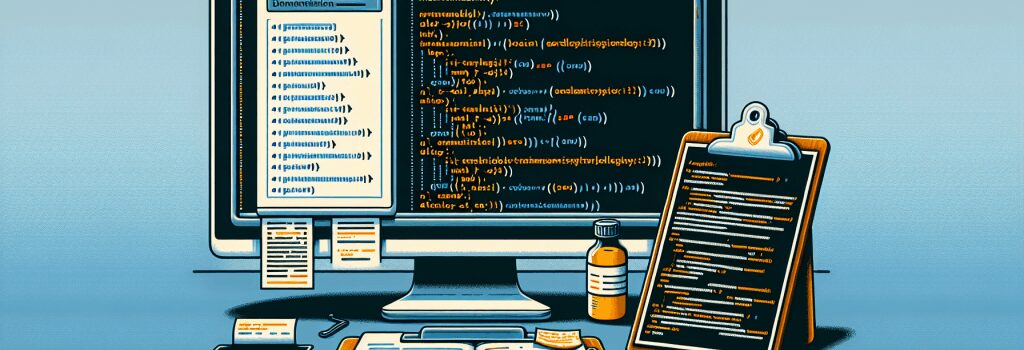
Maintaining code can be a challenge, and ensuring its readability is a critical part of sustainable development. PHP, a popular server-side scripting language used widely in web development, is no exception. In this section, we will discuss how to document PHP code properly for improved maintainability.
Why Good Documentation Matters
Documentation is an often overlooked but essential part of the development process. It not only helps others understand what your code does but also assists you in the future when you need to make updates or changes. Without good documentation, maintainability can become a nightmare, and time can be wasted trying to decipher what each function or class does.
Writing Descriptive Comments
When it comes to PHP code documentation, comments are your best friend. They enable you to explain in plain English what your code is doing. A good practice is to write descriptive comments for every function or class you develop. They should describe what the code does, any inputs it needs, the output it produces, and any side effects it may have.
Function Comments
Here is an example of function comment in PHP:
<pre><code>
/
Calculates the square of a number
@param int $num The number to be squared
@return int The squared result
/
function square($num) {
return $num $num;
}
Class Comments
Similar to function comments, class comments describe what the class is designed to do. If the class manipulates data or interacts with the database, these should be highlighted in the class comments.
<pre><code>
/
Class for handling user login
This class provides methods for user login, logout, and
check logged in status
*/
class UserLogin {
// class methods and properties go here
}
Using Proper Naming Conventions
Another critical part of documenting your PHP code is using meaningful and expressive variable, function, and class names. This helps to explain the purpose of the variable, function, or class which results in less confusion later on.
Variable Naming
Variable names should describe what they hold or the value they represent. For example, instead of naming a variable ;$d>, it would be much more informative to name it ;$distance>.
Function and Class Naming
Functions and classes should be named according to what they do. For example, a function that calculates the sum of two numbers might be named ;calculateSum()>. A class that is designed to handle user authentication might be called ;UserAuthentication>.
Implementing Code Formatting Standards
Lastly, maintaining a consistent code formatting style can greatly increase the readability and maintainability of your PHP code. This includes consistent indentation, consistent use of brackets, and proper spacing.
PSR Standards
The PHP community has agreed upon a set of coding standards known as the PHP Standards Recommendations (PSR). Following these conventions can enhance code readability and ease collaboration with other developers.
By following these guidelines, you can ensure that your PHP code is well-documented and maintainable. It might require a bit more effort initially, but the payoff in terms of reduced debugging and maintenance time is well worth it.
FAQ
Why is documenting PHP code important for maintainability?
Documenting PHP code is important for maintainability because it helps developers understand the purpose of the code, its functionality, and any potential pitfalls. This makes it easier for others (or even the original developer) to maintain or update the code in the future.
What are some best practices for documenting PHP code?
Some best practices for documenting PHP code include using clear and concise language, following a consistent format (such as PHPDoc comments), and providing examples when necessary to clarify complex logic.
What is PHPDoc and how can it be used to document PHP code?
PHPDoc is a documentation format that allows developers to document their PHP code using specific comment tags. By adding PHPDoc comments to functions, classes, and methods, developers can generate documentation automatically using tools like phpDocumentor.
How can well-documented PHP code improve collaboration within a development team?
Well-documented PHP code can improve collaboration within a development team by reducing confusion and misinterpretation of code. It allows team members to easily understand each other’s code and make changes or improvements more efficiently.
What are some common tools used for generating documentation from PHP code?
Some common tools used for generating documentation from PHP code include phpDocumentor, Doxygen, and ApiGen. These tools can parse PHP code and generate HTML or other formats of documentation based on PHPDoc comments.
How often should developers update documentation in PHP code?
Developers should update documentation in PHP code whenever there are significant changes to the codebase, such as adding new features, fixing bugs, or refactoring existing code. Regularly updating documentation ensures it remains accurate and useful.
How can comments be effectively used in PHP code to improve maintainability?
Comments in PHP code can be effectively used to explain the purpose of functions, classes, and methods, describe algorithms or complex logic, document assumptions or constraints, and provide warnings or TODO notes for future improvements.
What are the benefits of writing self-documenting PHP code?
The benefits of writing self-documenting PHP code include improved readability, reduced reliance on external documentation, easier maintenance, faster onboarding for new developers, and overall better code quality.
How can developers ensure that their PHP documentation remains up-to-date?
Developers can ensure that their PHP documentation remains up-to-date by incorporating documentation updates as part of their regular development process, using version control systems to track changes, reviewing and updating documentation during code reviews, and encouraging team members to contribute to documentation maintenance.
What are some common pitfalls to avoid when documenting PHP code?
Some common pitfalls to avoid when documenting PHP code include using vague or misleading comments, duplicating code information in comments, neglecting to update comments when code changes, and providing excessive or unnecessary documentation that adds little value.