Tips for Writing Clean and Maintainable PHP Code.
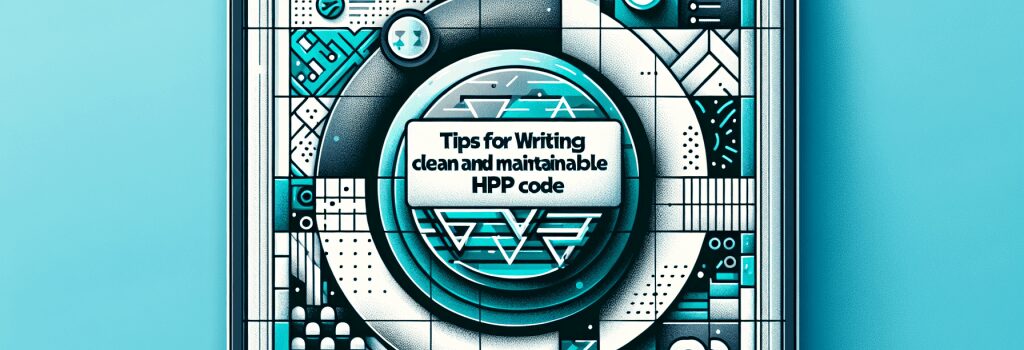
Introduction to Writing Clean and Maintainable PHP Code
Writing clean and maintainable PHP code is pivotal for any web developer, especially when working on larger projects or in team environments. PHP, being one of the most popular server-side scripting languages, offers tremendous flexibility. However, without adhering to good coding practices, this flexibility can lead to code that’s hard to read, maintain, or scale. This article aims to provide essential tips to help you write more structured, efficient, and maintainable PHP code.
Adopt a Consistent Naming Convention
One of the first steps towards maintaining clean code is to adopt and consistently follow a naming convention. Whether you choose camelCase or snake_case for your variables and functions, the key is consistency. This not only makes your code easier to read but also helps in identifying the purpose of variables and functions at a glance.
Use Comments Wisely
While comments are helpful, overly commented code or comments that state the obvious can clutter your codebase. Use comments to explain “why” something is done a certain way, not “what” is being done. Good code mostly speaks for itself. However, when working with complex logic or algorithms, well-placed comments can be invaluable.
Leverage PHP’s Built-in Functions
PHP comes with a vast library of built-in functions. Before writing your own function for a common task, check if there’s already a built-in function that can accomplish the same task. Using built-in functions not only saves time but also ensures that your application is optimized and maintains a certain standard of reliability.
Practice Object-Oriented Programming (OOP)
Whenever possible, use object-oriented programming (OOP) practices. OOP helps in organizing your code better, making it easier to understand, maintain, and extend. Concepts like classes, objects, inheritance, and polymorphism encourage the reuse of code and the principle of encapsulation helps in safeguarding the data within your application.
Follow the Principles of Clean Code
Use Meaningful Names
Choose variable, function, and class names that clearly express their intention and scope. Avoid using generic names like ;data> or ;info>, and opt for more descriptive names that convey the purpose.
Keep Functions and Classes Short
The Single Responsibility Principle (SRP) states that a function or class should only have one reason to change. This implies keeping your functions focused on a single task and your classes on a single concept. It’s easier to read, test, and debug smaller chunks of code.
Refactor Repeated Code
Don’t repeat yourself (DRY) is a principle aimed at reducing repetition within code. If you find yourself writing the same or very similar code in multiple places, consider refactoring that code into a single function or class method.
Invest Time in Learning Design Patterns
Design patterns are standardized solutions to common problems in software design. Familiarizing yourself with patterns like Factory, Singleton, or Observer can help you write more maintainable code. These patterns offer templates for structuring your code in ways that make it scalable, easier to understand, and more adaptable to change.
Utilize Version Control
Version control systems, like Git, are not directly related to clean code but play a crucial role in maintaining the history of your code changes. With version control, you can experiment with new features or changes without the risk of losing your previous work. It also simplifies collaboration with others by allowing multiple developers to work on the same project simultaneously.
Conclusion
Writing clean and maintainable PHP code is a skill that improves with practice and discipline. By adhering to the tips mentioned above, developers can ensure that their codebase is not only functional but also clean, efficient, and scalable. Remember, the goal is to write code that your future self and your peers can understand and work with, without having to decipher it anew. Happy coding!