Implementing User Authentication in PHP
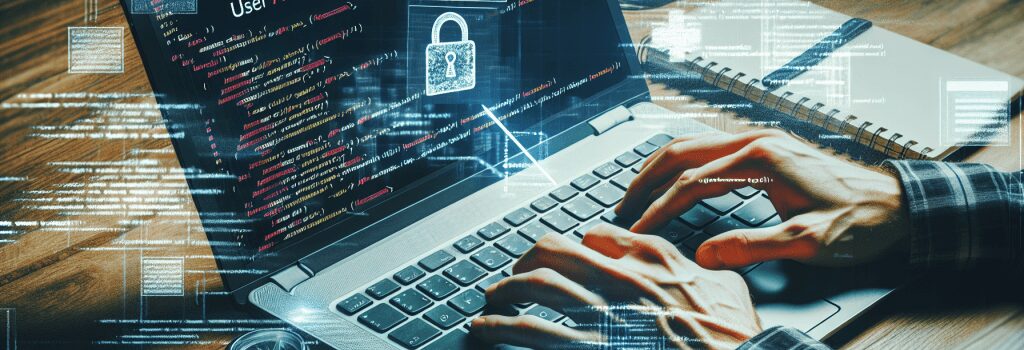
Understanding User Authentication in PHP
User authentication is a critical component of web development, ensuring that only authorized users can access certain resources. PHP, a server-side scripting language, provides various methods to implement secure user authentication. This article dives into the basics of creating a user authentication system using PHP, highlighting best practices to keep user data secure.
What is User Authentication?
User authentication is a process that verifies if an individual or entity is who they claim to be. In web development, this typically involves checking a username and password against stored data. If the credentials match, the system grants access to protected resources.
Setting Up the Environment
Before we begin, ensure your development environment has PHP and a MySQL database set up. User credentials will be stored in the database, which PHP scripts will access to authenticate users.
Step 1: Creating the Database
First, create a database to store user details. Use the following SQL command to create a table named ;users>:
sql
CREATE TABLE users (
id INT(11) NOT NULL AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
password VARCHAR(255) NOT NULL
);
Remember to hash passwords using PHP’s ;password_hash()> function before storing them to ensure security.
Step 2: User Registration
Create a PHP script for user registration. This script should collect user credentials and store them in the database. Here’s a simple example:
php
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
include 'db_connect.php'; // Assume this file establishes database connection
$username = $_POST['username'];
$password = password_hash($_POST['password'], PASSWORD_DEFAULT);
$stmt = $conn->prepare("INSERT INTO users (username, password) VALUES (?, ?)");
$stmt->bind_param("ss", $username, $password);
$stmt->execute();
echo "Registration successful!";
}
?>
Step 3: User Login
Now, let’s handle user login. The login script should verify if the entered credentials match those stored in the database.
php
<?php
session_start();
if ($_SERVER["REQUEST_METHOD"] == "POST") {
include 'db_connect.php';
$username = $_POST['username'];
$password = $_POST['password'];
$stmt = $conn->prepare("SELECT id, username, password FROM users WHERE username = ?");
$stmt->bind_param("s", $username);
$stmt->execute();
$result = $stmt->get_result();
if ($result->num_rows > 0) {
$row = $result->fetch_assoc();
if (password_verify($password, $row['password'])) {
$_SESSION['loggedin'] = true;
$_SESSION['username'] = $row['username'];
echo "Login successful!";
} else {
echo "Incorrect password.";
}
} else {
echo "Username does not exist.";
}
}
?>
Step 4: Session Management
After successful login, use PHP sessions to maintain the user’s logged-in state across pages. Call ;session_start()> at the beginning of each script that requires user authentication.
Step 5: Logout
To log out, simply destroy the session using ;session_destroy()> and unset any session variables.
php
<?php
session_start();
session_unset();
session_destroy();
header("Location: login.php");
exit();
?>
Security Practices
– Always hash passwords using PHP’s ;password_hash()> and verify with ;password_verify()>.
– Use HTTPS to encrypt data transmitted over the network.
– Sanitize and validate all user inputs to prevent SQL injection and other attacks.
Implementing user authentication in PHP is a foundational skill for web developers. By following the steps outlined in this article and adhering to security best practices, you can create a secure and efficient authentication system for your web applications.