Utilizing JavaScript Generators and Iterators for Advanced Data Handling
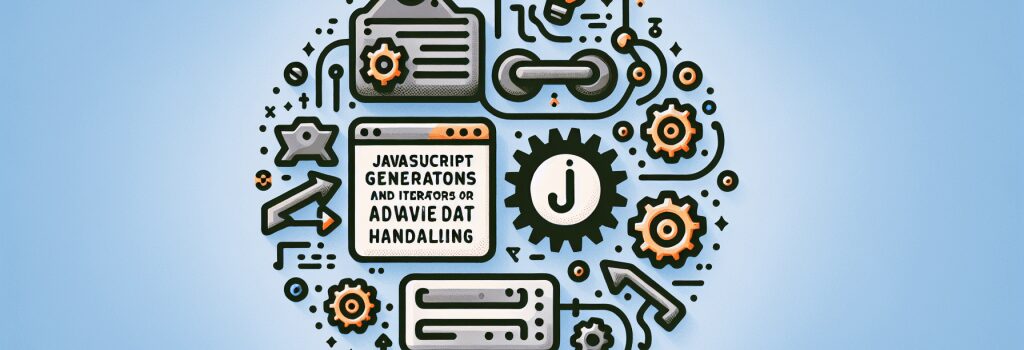
We’ve got something fun on the menu today; JavaScript Generators and Iterators! But don’t worry, they’re not like those intimidating maths symposia, and no one’s asking you to disprove Fermat’s last theorem here! Grab a coffee, sit back, relax, and let’s take this ride through the intricacies of advanced data handling in JavaScript together.
What are JavaScript Generators and Iterators?
Let’s start at the beginning. Imagine you’re at a comedy show. The comedian (let’s call him Bob) cracks a joke, waits for the audience to laugh, and then moves to the next joke. Generators are just like good ol’ Bob! They are super polite, pause after delivering a chunk of calculation (a joke in Bob’s case), and resume when you are ready for the next punchline.
On the other hand, an Iterator is like a finicky book reader. Instead of reading the whole book (Array) at once, our Iterator takes it one page (element) at a time, until it finishes or gets bored (runs out of elements). In technical jargon, an Iterator is an object that allows you to traverse through an iterable object (like an array or a string), one element at a time.
Why Do We Need Them?
Well, while it’d be amazing if real life was a straight journey with no hiccups or surprises, in the digital world of programming we do love challenges, don’t we?
Generators and Iterators make it possible to handle large amounts of data without tanking your application’s performance. Plus, they make your code cleaner and more maintainable.
Imagine if Bob had to spill all his jokes at once or if our reader had to read through the whole book without breaks – chaos, right? That’s what Generators and Iterators help avoid in your code.
Deal with Generators
Creating a generator is as simple as making a cup of coffee, well, almost. You just need to add an asterisk (*) after the ;function> keyword, which signals that it’s not just a regular function, it’s a ‘pause-able’ function.
Here, ;yield> is like Bob waiting for the laughter to die down. Each time a ;yield> is encountered, the function pauses, kind of like pausing your favorite Netflix series between cliffhangers.
Interacting with Generators
Interacting with a generator is like dealing with a vending machine. You put in a coin (call ‘next()’), and out comes a soda (the ‘yielded’ value).
Iterators At Work
Think of an iterator as your transparent window to an inventory, say your bookshelf. You oversee one book at a time starting from the left, going right, without knowing what book you pick until you pick it. An iterator is an object created by invoking the ;[Symbol.iterator]()> method on an iterable object.
In the example above, each call to ;next()> gives the next book from the ‘shelf’.
And voila! That’s the story of JavaScript Generators and Iterators, two very important programming concepts. Next time you face a massive data management task, remember the comedian Bob, the finicky reader, and their real-life JavaScript counterparts. Happy coding!