JavaScript Asynchronous Programming: Callbacks, Promises, and Async/Await
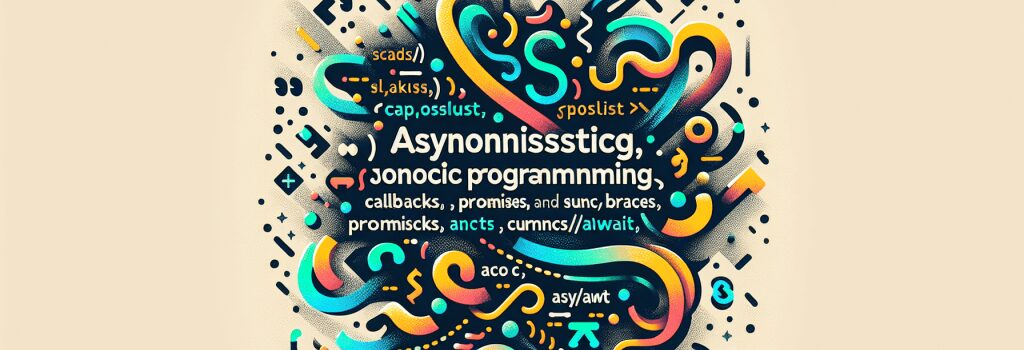
Understanding JavaScript Asynchronous Programming
JavaScript asynchronous programming is a fundamental concept for developing modern web applications. Unlike traditional synchronous programming, where operations are completed one at a time, asynchronous programming allows multiple operations to run concurrently, improving the performance and usability of web applications. This article explores the three pillars of asynchronous programming in JavaScript: callbacks, promises, and async/await.
Callbacks: The Backbone of Asynchronous Programming
Callbacks are functions passed as arguments to other functions. They allow you to defer certain operations until a previous operation has been completed. This technique is particularly useful in handling operations like reading files or making API calls, which might take some time to complete.Example of a Callback Function:
Although callbacks are a step forward in handling asynchronous tasks, they have limitations, particularly when managing multiple asynchronous operations, leading to a situation often termed "Callback Hell" or "The Pyramid of Doom".
Promises: Escaping Callback Hell
To address the complexities and limitations of callbacks, Promises were introduced in ES6. A Promise is an object representing the eventual completion or failure of an asynchronous operation. Promises allow you to attach callbacks, rather than passing them, and they significantly simplify error handling with ;.then()> for success and ;.catch()> for failure.Basic Promise Usage:
Async/Await: Syntactic Sugar for Promises
Introduced in ES8, async/await offers a cleaner, more readable syntax for working with promises. An ;async> function always returns a promise, and the ;await> keyword pauses the execution of the function until the awaited Promise is resolved.
Example of Async/Await:
Handling Errors in Async/Await
To handle errors in async/await, you can use the traditional try/catch block, making error handling cleaner and more straightforward.
Conclusion
Understanding and effectively using callbacks, promises, and async/await are crucial for any web developer working with JavaScript. These constructs not only improve the readability and maintenance of your code but also enhance the performance and user experience of your web applications. As you progress in your development career, mastering asynchronous programming in JavaScript will undoubtedly be a valuable skill in your toolkit.