Implementing AJAX in Your Web Application with Vanilla JavaScript
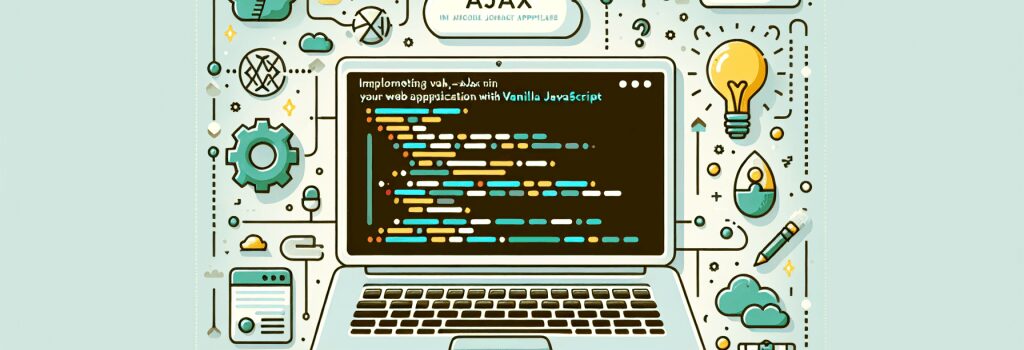
Introduction to AJAX in Web Development
AJAX, which stands for Asynchronous JavaScript and XML, is a vital technology for creating fast and dynamic web applications. It allows for the exchange of data with a server and updating parts of a web page without reloading the whole page. This article explores how to implement AJAX in your web applications using Vanilla JavaScript, a crucial skill for any aspiring web developer.
Understanding the Basics of AJAX
Before diving into the implementation, it’s essential to grasp the foundation of AJAX. AJAX is not a programming language but a technique used in web development to create interactive applications. It works by sending and receiving data asynchronously between a web page’s front end and a server. This process enhances the user experience by making web applications faster and more responsive.
Setting Up Your Environment for AJAX
To start working with AJAX, you first need to set up a simple web development environment. This involves having a basic understanding of HTML, CSS, and JavaScript. You will also need a simple server setup; for testing purposes, local servers like XAMPP or WampServer will do just fine. Ensure your project directory is correctly set up within your server’s root folder to avoid any path-related issues.
Implementing AJAX with Vanilla JavaScript
Creating the XMLHttpRequest Object
The core of AJAX in Vanilla JavaScript is the ;XMLHttpRequest> object. This object allows you to make HTTP requests to retrieve data from a server asynchronously.
Establishing a Connection
Next, you must establish a connection to the server using the ;open()> method of the ;XMLHttpRequest> object. This method takes three primary parameters: the request method (GET, POST, etc.), the URL, and a boolean value indicating whether the request should be handled asynchronously.
Sending the Request
After establishing a connection, you can send the request to the server using the ;send()> method. For a GET request, you don’t need to pass any parameters to ;send()>.
Handling the Response
Handling the server’s response is done by setting up an ;onreadystatechange> event handler on the ;XMLHttpRequest> object. This handler checks the ;readyState> property of the request; when it equals ;4> (which means the request has completed), and the ;status> is ;200> (indicating a successful HTTP request), you can process the returned data.
Making an AJAX Call with POST Method
While the GET method is straightforward, using the POST method requires sending data as a parameter of the ;send()> method. This is particularly useful for submitting forms asynchronously.
Utilizing AJAX Response Data
Once you have the data from the server, you can manipulate the DOM to reflect the changes on your web page. For example, if you’re fetching data that returns JSON, you can parse this data and dynamically create HTML elements based on it.
Conclusion: Enhancing User Experience with AJAX
Implementing AJAX in your web applications using Vanilla JavaScript is an effective way to improve user experience by making your site faster and more interactive. By following the steps outlined in this guide, you can start integrating AJAX into your projects, enhancing your skills as a web developer and making your applications more dynamic and engaging for users. Remember, practice and experimentation are key to mastering AJAX, so don’t hesitate to explore and build upon the basics covered here.