Selecting Elements with JavaScript: A Beginner’s Guide
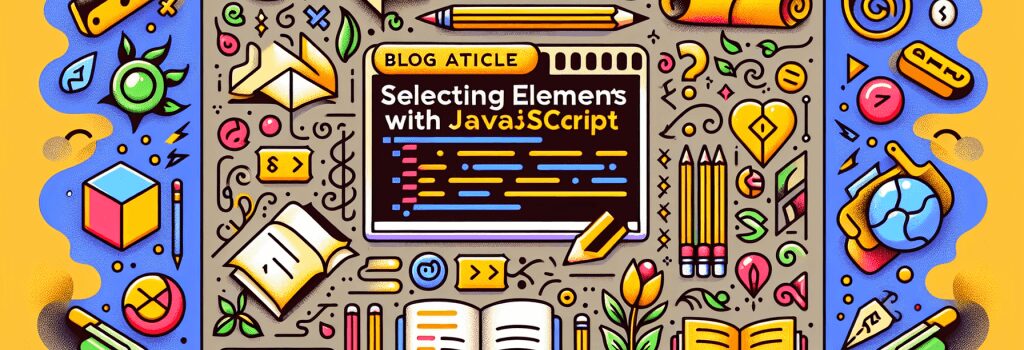
Selecting elements with JavaScript is a crucial skill for any aspiring web developer. It allows you to dynamically interact with, modify, and update the HTML elements of a webpage, making your web applications more interactive and responsive. This guide will walk you through the basics of selecting elements with JavaScript, using various methods to access the DOM (Document Object Model).
Understanding the Document Object Model (DOM)
Before diving into selecting elements, it’s important to have a basic understanding of the DOM. The DOM is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as nodes and objects; this way, programming languages can interact with the webpage.
Selecting Elements by ID
One of the simplest ways to select an element is by its ID. Each element can have a unique ID attribute, which you can use to select it directly. JavaScript provides the ;getElementById()> method for this purpose.
javascript
const element = document.getElementById('example-id');
This method will return the element with the specified ID. If no element with that ID exists, it returns ;null>.
Selecting Elements by Class Name
Often, you might want to select multiple elements that share the same class. JavaScript offers the ;getElementsByClassName()> method for this.
javascript
const elements = document.getElementsByClassName('example-class');
This method returns a live HTMLCollection of all elements with the specified class name. Remember, since it returns a collection, you might need to loop through the items or access them by index.
Selecting Elements by Tag Name
If you want to select elements based on their tag name (for example, all ;<div>> elements), you can use the ;getElementsByTagName()> method.
javascript
const elements = document.getElementsByTagName('div');
Similar to ;getElementsByClassName()>, this method returns a live HTMLCollection of elements.
Query Selector
For more complex selections, the ;querySelector()> and ;querySelectorAll()> methods allow you to select elements using CSS selectors. The ;querySelector()> method returns the first element that matches the specified selector.
javascript
const element = document.querySelector('.example-class');
The ;querySelectorAll()> method returns a NodeList of all elements matching the specified selector.
javascript
const elements = document.querySelectorAll('.example-class');
These methods provide a powerful way to select elements, as they can use any CSS selector, including combinations, pseudo-classes, and attributes.
Best Practices
When selecting elements, it’s crucial to ensure that the DOM is fully loaded. Attempting to select elements before the DOM is fully loaded can result in errors or elements not being found. To avoid this, place your script tags at the bottom of the body or use event listeners to run your code only after the DOM has fully loaded.
javascript
document.addEventListener('DOMContentLoaded', function() {
// Your code to select elements here
});
Conclusion
Selecting elements is a foundational skill in web development, allowing you to manipulate and interact with the webpage dynamically. By understanding and utilizing the different methods JavaScript provides, you can select any element on the page efficiently. Remember to practice these methods and experiment with different selectors to become proficient in manipulating the DOM.