JavaScript Best Practices for Writing Clean Conditional Statements
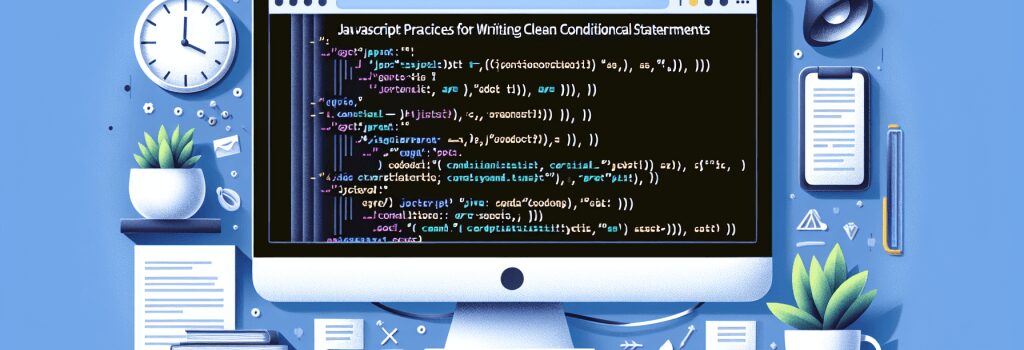
JavaScript Best Practices for Writing Clean Conditional Statements
Writing clean, efficient, and maintainable code is a hallmark of a skilled web developer. Conditional statements, such as ;if>, ;else if>, and ;switch>, play a critical role in decision-making processes within your JavaScript applications. By adhering to best practices, you can ensure your conditional statements enhance the readability, performance, and reliability of your code. Here are some crucial guidelines to follow when crafting your conditional statements in JavaScript.
Understand When to Use ;if-else> vs. ;switch>
Choosing Between ;if-else> and ;switch>
Conditional statements often start simple but can become complex and nested over time. Understanding the appropriate scenarios for using ;if-else> versus ;switch> can significantly impact your code’s clarity and performance.
– Use ;if-else> for comparisons involving ranges or non-equal conditions.
– Opt for ;switch> when dealing with multiple conditions that test equality against the same variable. It not only makes the code more readable but can also be more efficient in certain JavaScript engines.
Simplify Conditions
Avoiding Unnecessary Complexity
Complex conditions can make your code hard to read and understand. Simplify your conditions by:
– Avoiding negative conditions when possible. They can be harder to grasp than positive ones.
– Using boolean variables to store condition results for clarity.
– Leveraging truthy and falsy values in JavaScript to streamline your code, but be mindful of values like ;0>, ;”>, ;null>, and ;undefined>, which can lead to unexpected outcomes if not used carefully.
Decouple Complex Conditions
Breaking Down Complex Logical Expressions
When faced with a condition that involves multiple logical operators, consider breaking it down into smaller, more manageable pieces. This approach not only enhances readability but also makes debugging easier.
Use Ternary Operators for Simple Conditions
Leveraging Conciseness with Ternary Operators
Ternary operators (;condition ? exprIfTrue : exprIfFalse>) offer a concise way to express simple ;if-else> statements. They are particularly useful for assignments and return statements. However, overusing ternary operators or nesting them can lead to code that’s hard to read, so use them judiciously.
Early Return Pattern
Reducing Nesting through Early Returns
Nesting can quickly lead to the dreaded “arrow code” where your code drifts to the right, making it hard to follow. Employ the early return pattern to handle conditions that don’t meet your function’s main purpose early on. This strategy not only reduces nesting but also minimizes the cognitive load for readers.
Use Meaningful Variable Names
Enhancing Readability with Descriptive Variables
The names you choose for your variables can significantly affect your code’s readability. Use meaningful, descriptive names for variables that hold condition results. This practice makes your conditions easier to understand at a glance.
Regularly Refactor
Keeping Conditions Clean through Refactoring
As your application evolves, so should your conditional statements. Regularly review and refactor your conditions to apply newer, cleaner, and more efficient practices. This continuous improvement helps maintain the quality and readability of your codebase.
Conclusion
Conditional statements are a foundation of JavaScript programming. By applying these best practices, you can write conditions that not only work efficiently but are also easy to read and maintain. Remember, clean code is not just about performance—it’s also about making your code accessible to others, including your future self.
Incorporating these guidelines into your daily coding practice will help you become a more proficient and sought-after web developer.